Introduction
Computer vision has revolutionized numerous industries, from security surveillance and autonomous vehicles to healthcare and retail. Among the most effective object detection algorithms is YOLO (You Only Look Once), a state-of-the-art model known for its speed and accuracy. With the upcoming YOLO 11 release, object detection is set to reach new heights, providing enhanced accuracy, reduced latency, and greater adaptability to complex scenes.
In this guide, we will discuss how to use YOLO 11 to build a computer vision model capable of detecting specific objects in a camera video feed. We will cover key concepts, setup, implementation, optimization techniques, and best practices for real-world applications.
What is YOLO 11?
YOLO 11 is the latest iteration of the YOLO object detection model, improving upon its predecessors in terms of:
Higher accuracy: Enhanced model architecture improves detection precision.
Lower inference time: Optimized computations lead to faster object recognition.
Improved adaptability: Supports various environments and complex scenarios with better generalization.
Better handling of small objects: Advanced feature extraction allows for more reliable detection of smaller objects.
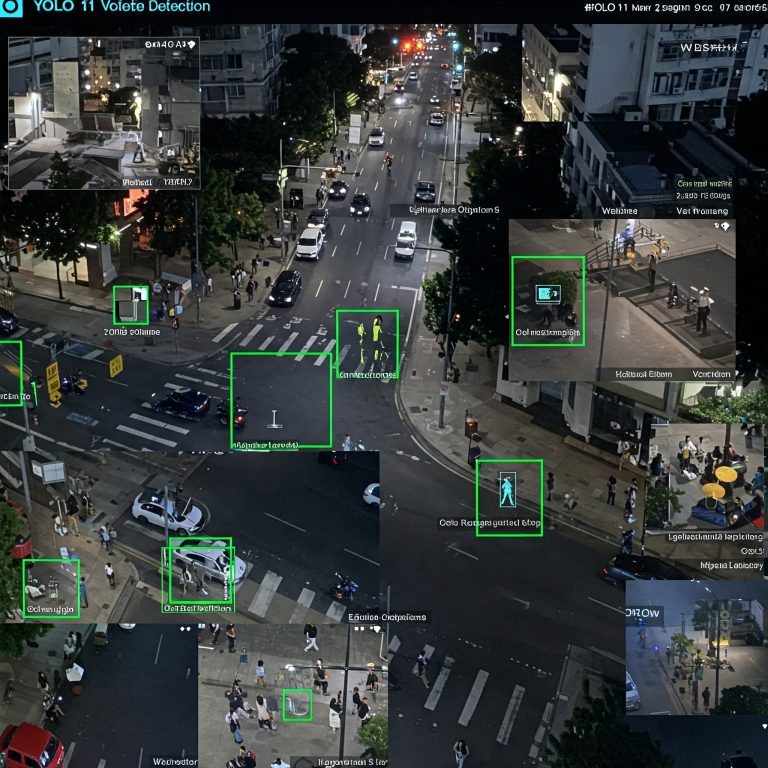
Setting Up the Environment
Before implementing YOLO 11, we need to set up our development environment. Follow these steps:
Prerequisites
Install Python (>=3.8)
sudo apt update && sudo apt install python3 python3-pip
Install essential libraries
pip install torch torchvision opencv-python numpy matplotlib
Download YOLO 11 Pretrained Weights
Visit the official YOLO 11 repository.
Download the model weights (
yolo11.weights
).Store them in your working directory.
Setting Up OpenCV for Video Processing
OpenCV will be used to handle video input and processing:
import cv2
import torch
import numpy as np
from yolov11 import YOLO11 # Import the YOLO 11 model
# Load the pre-trained model
model = YOLO11(weights='yolo11.weights')
model.eval()
Â
Implementing YOLO 11 for Object Detection
Once the setup is complete, we can integrate YOLO 11 with a real-time camera feed to detect specific objects.
Loading a Video Stream
cap = cv2.VideoCapture(0) # Capture from webcam
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# Convert frame to tensor
img_tensor = torch.from_numpy(frame).permute(2, 0, 1).float().unsqueeze(0)
# Perform inference
results = model(img_tensor)
# Process detection results
for box in results:
x1, y1, x2, y2, label, confidence = box
cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.putText(frame, f'{label} {confidence:.2f}', (x1, y1 - 5),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow('YOLO 11 Object Detection', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Optimizing Object Detection
To improve the accuracy and speed of our model, consider:
Adjusting input size: Larger input sizes improve detection accuracy but increase computational load.
Fine-tuning confidence thresholds: Setting an optimal confidence threshold prevents false positives.
Using batch inference: Processing multiple frames in a batch optimizes inference speed.
Applications of YOLO 11 in Object Detection
1. Security Surveillance
Real-time object detection can identify unauthorized individuals, weapons, or suspicious objects in surveillance footage.
2. Autonomous Vehicles
YOLO 11 can be integrated into self-driving systems to detect pedestrians, vehicles, and traffic signs for safer navigation.
3. Retail and Inventory Management
Stores can use YOLO 11 for automated checkout systems, shelf monitoring, and detecting missing products in stock.
4. Industrial Automation
Factories can leverage object detection to monitor machinery, detect defects, and ensure worker safety.
5. Medical Imaging
YOLO 11 can assist in detecting abnormalities in X-rays, MRIs, and CT scans, improving diagnostic accuracy.
Future of YOLO-Based Object Detection
The future of object detection with YOLO looks promising, with continuous improvements in accuracy, efficiency, and deployment flexibility. As deep learning models evolve, we can expect even better real-time performance, edge computing compatibility, and AI-powered analytics that integrate seamlessly into various applications.
Conclusion
YOLO 11 presents an advanced and efficient solution for real-time object detection in video feeds. With its powerful deep learning capabilities, this model enables developers to create sophisticated computer vision applications in diverse industries. By leveraging YOLO 11’s speed, accuracy, and scalability, we can enhance security, automation, and accessibility across multiple domains.
Start Learning Python Now
If you would like to learn how to use YOLO someday, why not start with learning the Python programming language now. You can register on our (currently) free course. It introduces you to the basic concepts that you will need to jump onto the bandwagon of computer programming, and especially, into the exciting world of Artificial Intelligence (AI) and Computer Vision.
Start small, take your time and learn the concepts. That’s how we started, and it paid off. It paid off by enabling us to assimilate and entrench the basic concepts that we need for learning to code.
You can register, log in, read through the concepts, read the exercises and solutions, and take a break (quite important when learning programming). You would also be able to call attention to the expert tutor to give you some guidelines. Get onto the course now by clicking on this link Python for Beginners
References
Redmon, J., & Farhadi, A. (2018). “YOLOv3: An Incremental Improvement.” arXiv preprint arXiv:1804.02767.
Bochkovskiy, A., Wang, C. Y., & Liao, H. Y. M. (2020). “YOLOv4: Optimal Speed and Accuracy of Object Detection.” arXiv preprint arXiv:2004.10934.
Jocher, G., et al. (2023). “YOLOv8: A Comprehensive Review and Implementation.” Ultralytics Documentation.
OpenCV Documentation: https://docs.opencv.org
PyTorch Documentation: https://pytorch.org/docs/stable/index.html